import java.applet.Applet;
import java.util.*;
import java.awt.*;
import java.awt.event.*;
public class sample1 extends Applet implements Runnable{
Thread thread=null;
Image bufImg;
Graphics gr;
int hour;
int minute;
int second;
public void init(){
bufImg=createImage(getWidth(),getHeight());
gr=bufImg.getGraphics();
setBackground(Color.white);
time();
}
public void start(){
if(thread==null){
thread=new Thread(this);
thread.start();
}
}
public void stop(){
thread=null;
}
public void paint(Graphics g){
gr.clearRect(0,0,getWidth(),getHeight());
gr.setFont(new Font("System",Font.BOLD,14));
gr.drawString("現在の時刻:"+hour+"時"+minute+"分"+second+"秒",10,20);
g.drawImage(bufImg,0,0,this);
}
public void update(Graphics g){
paint(g);
}
public void run(){
while(thread!=null){
time();
repaint();
try{
thread.sleep(100);
}catch(InterruptedException e){
e.printStackTrace();
}
}
}
public void time(){
Calendar cl;
cl=Calendar.getInstance(TimeZone.getTimeZone("JST"));
hour=cl.get(Calendar.HOUR);
minute=cl.get(Calendar.MINUTE);
second=cl.get(Calendar.SECOND);
}
}
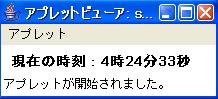
|